本文參考文章,出于學(xué)習(xí)目的,寫本文。
開發(fā)項目時,為了維護(hù)一些經(jīng)常需要變更的數(shù)據(jù),比如數(shù)據(jù)庫的連接信息、請求的url、測試數(shù)據(jù)等,需要將這些數(shù)據(jù)寫入配置文件,將數(shù)據(jù)和代碼分離,只需要修改配置文件的參數(shù),就可以快速完成環(huán)境的切換或者測試數(shù)據(jù)的更新,常用的配置文件格式有ini、json、yaml等,下面簡單給大家介紹下,Python如何讀寫這幾種格式的文件。
1、ini格式
ini 即 Initialize ,是Windows中常用的配置文件格式,結(jié)構(gòu)比較簡單,主要由節(jié)(Section)、鍵(key)和值(value)組成。每個獨(dú)立部分稱之為section,每個section內(nèi),都是key(option)=value形成的鍵值對。
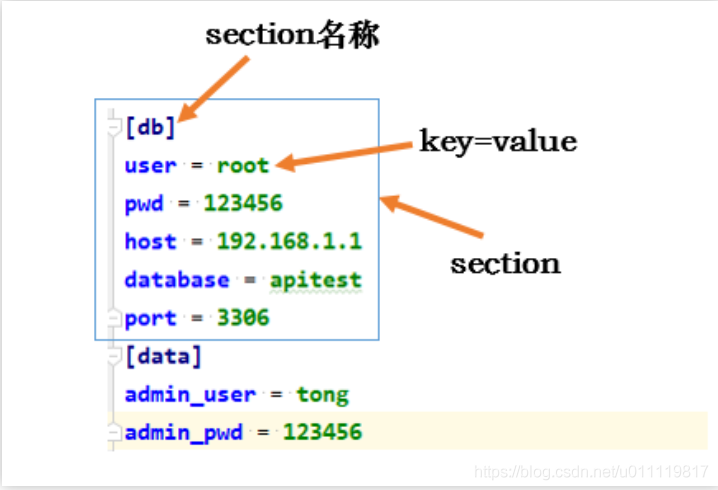
在Python3中,使用自帶的configparser庫(配置文件解析器)來解析類似于ini這種格式的文件,比如config、conf。
可以看到,ini只有字典一種格式,且全部都是字符串。
1.1 ini的讀取刪除操作
import configparser
#使用前,需要創(chuàng)建一個實例
config = configparser.ConfigParser()
#讀取并打開文件
config.read('test.ini',encoding='utf-8')
#獲取sections,返回列表
print(config.sections())
#[db,data]
#獲取sections下的所有options
print(config.options('db'))
#['user', 'pwd', 'host', 'database', 'port']
#獲取指定section下指定的options
print(config.get('db','user'))
# root
#獲取section中所有鍵值對
print(config.items('data'))
#[('admin_user', 'tong'), ('admin_pwd', '123456')]
#刪除整個section
config.remove_section('data')
#刪除某個section下的key
config.remove_option('db','host')
print(config.items('db'))
1.2 ini 寫入操作
寫入操作可能會比較少
import configparser
config=configparser.ConfigParser()
config['url']={'url':'www.baidu.com'} #類似于字典操作
with open('example.ini','w') as configfile:
config.write(configfile)

2.JSON格式
JSON (JavaScript Object Notation) 是一種輕量級的數(shù)據(jù)交換格式,采用完全獨(dú)立于語言的文本格式,這些特性使json成為理想的數(shù)據(jù)交換語言,易于閱讀和編寫,同時易于機(jī)器解析和生成。
2.1 JSON示例格式
{
"name":"smith",
"age":30,
"sex":"男"
}
Python中使用內(nèi)置模塊json操作json數(shù)據(jù),使用json.load()和json.dump方法進(jìn)行json格式文件讀寫:
# 讀取json
import json
with open('test1.json') as f:
a = json.load(f)
print(a)
# 寫入json
import json
dic ={
"name" : "xiaoming",
"age" : 20,
"phonenumber" : "15555555555"
}
with open("test2.json", "w") as outfile:
json.dump(dic, outfile)
有關(guān)json更多的介紹請看鏈接
3. yaml格式
yaml全稱Yet Another Markup Language(另一種標(biāo)記語言),它是一種簡潔的非標(biāo)記語言,以數(shù)據(jù)為中心,使用空格,縮進(jìn),分行組織數(shù)據(jù),解析成本很低,是非常流行的配置文件語言。
3.1 yaml的語法特點(diǎn)
- 大小寫敏感
- 使用縮進(jìn)表示層級關(guān)系,縮進(jìn)的空格數(shù)目不重要,只要相同層級的元素左側(cè)對齊即可
- 縮進(jìn)時不允許使用Tab鍵,只允許使用空格。
- 字符串不需要使用引號標(biāo)注,但若字符串包含有特殊字符則需用引號標(biāo)注
- 注釋標(biāo)識為#
3.2 yaml示例
case1:
info:
title: "正常登陸"
url: http://192.168.1.1/user/login
method: "POST"
json:
username: "admin"
password: "123456"
expected:
status_code:
- 200
- 300
content: "user_id"
讀取后效果:

yaml支持的數(shù)據(jù)結(jié)構(gòu)有三種
- 對象:鍵值對的集合,又稱為映射(mapping)/ 哈希(hashes) / 字典(dictionary)
- 數(shù)組:一組按次序排列的值,又稱為序列(sequence) / 列表(list)
- 純量(scalars):單個的、不可再分的值。字符串、布爾值、整數(shù)、浮點(diǎn)數(shù)、Null、時間、日期
Python中使用pyyaml處理yaml格式數(shù)據(jù)
使用前,需要進(jìn)行安裝:
3.3 yaml文件讀取
用python讀取yaml文件,先用open方法讀取文件數(shù)據(jù),再通過load方法轉(zhuǎn)成字典。
import yaml
with open("testyaml.yaml", encoding='utf-8') as file:
data = yaml.safe_load(file)
print(data)
print(data['case1']['json'])
print(data['case1']['json']['username'])
3.4 yaml文件的寫入
import yaml
#定義一個字典
content = {
'id': 1,
'text': 'programming languages',
'members': ['java', 'python', 'python', 'c', 'go', 'shell'],
'next': {'a':1,'b':2}
}
with open('test3.yaml', 'w', encoding='utf-8') as file:
yaml.dump(content, file, default_flow_style=False, encoding='utf-8', allow_unicode=True)
以上有三種數(shù)據(jù)類型,寫入文件效果為:
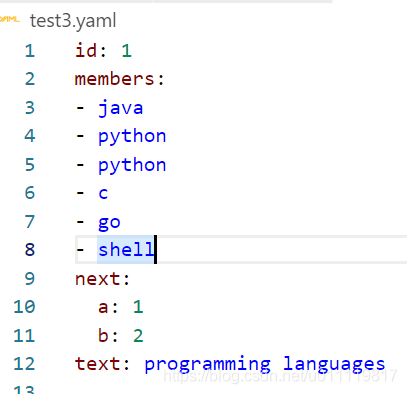
當(dāng)然手動寫也沒有問題。
到此這篇關(guān)于Python常用配置文件ini、json、yaml讀寫總結(jié)的文章就介紹到這了,更多相關(guān)Python讀寫ini、json、yaml配置文件內(nèi)容請搜索腳本之家以前的文章或繼續(xù)瀏覽下面的相關(guān)文章希望大家以后多多支持腳本之家!
您可能感興趣的文章:- Python中rapidjson參數(shù)校驗實現(xiàn)
- Python中json.load()和json.loads()有哪些區(qū)別
- Python中json.dumps()函數(shù)的使用解析
- python 存儲json數(shù)據(jù)的操作
- 解決python3 json數(shù)據(jù)包含中文的讀寫問題
- python json.dumps中文亂碼問題解決
- Python如何把不同類型數(shù)據(jù)的json序列化
- python之json文件轉(zhuǎn)xml文件案例講解