動(dòng)態(tài)網(wǎng)頁(yè)爬取是爬蟲(chóng)學(xué)習(xí)中的一個(gè)難點(diǎn)。本文將以知名插畫(huà)網(wǎng)站pixiv為例,簡(jiǎn)要介紹動(dòng)態(tài)網(wǎng)頁(yè)爬取的方法。
寫(xiě)在前面
本代碼的功能是輸入畫(huà)師的pixiv id,下載畫(huà)師的所有插畫(huà)。由于本人水平所限,所以代碼不能實(shí)現(xiàn)自動(dòng)登錄pixiv,需要在運(yùn)行時(shí)手動(dòng)輸入網(wǎng)站的cookie值。
重點(diǎn):請(qǐng)求頭的構(gòu)造,json文件網(wǎng)址的查找,json中信息的提取
分析
創(chuàng)建文件夾
根據(jù)畫(huà)師的id創(chuàng)建文件夾(相關(guān)路徑需要自行調(diào)整)。
def makefolder(id): # 根據(jù)畫(huà)師的id創(chuàng)建對(duì)應(yīng)的文件夾
try:
folder = os.path.join('E:\pixivimages', id)
os.mkdir(folder)
return folder
except(FileExistsError):
print('the folder exists!')
exit()
獲取作者所有圖片的id
訪問(wèn)url:https://pixiv.net/ajax/user/畫(huà)師id/profile/all(這個(gè)json可以在畫(huà)師主頁(yè)url:https://www.pixiv.net/users/畫(huà)師id 的開(kāi)發(fā)者面板中找到,如圖:)
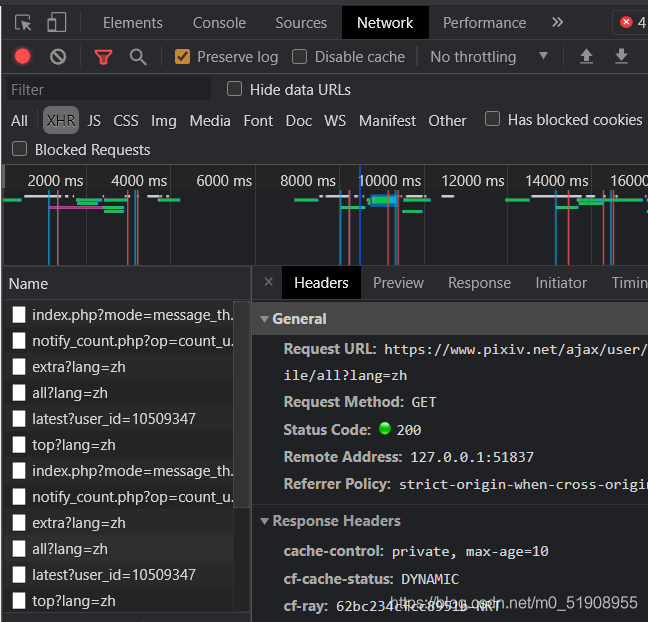
json內(nèi)容:
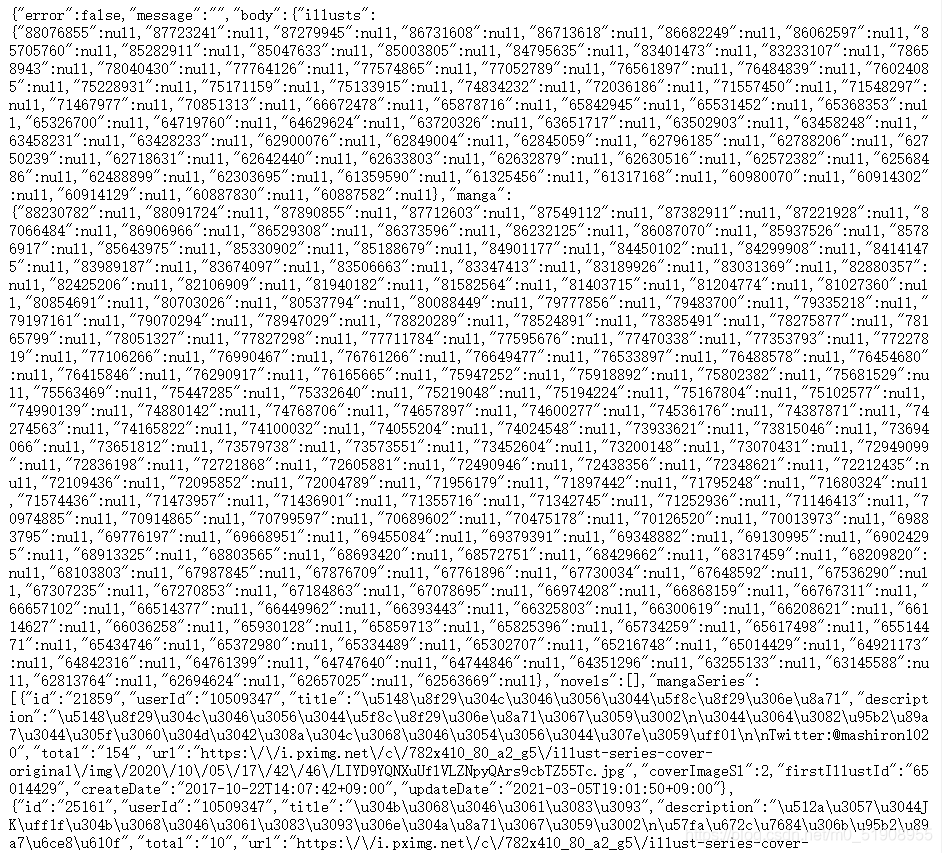
將json文檔轉(zhuǎn)化為python的字典,提取對(duì)應(yīng)元素即可獲取所有的插畫(huà)id。
def getAuthorAllPicID(id, cookie): # 獲取畫(huà)師所有圖片的id
url = 'https://pixiv.net/ajax/user/' + id + '/profile/all' # 訪問(wèn)存有畫(huà)師所有作品
headers = {
'User-Agent': user_agent,
'Cookie': cookie,
'Referer': 'https://www.pixiv.net/artworks/'
# referer不能缺少,否則會(huì)403
}
res = requests.get(url, headers=headers, proxies=proxies)
if res.status_code == 200:
resdict = json.loads(res.content)['body']['illusts'] # 將json轉(zhuǎn)化為python的字典后提取元素
return [key for key in resdict] # 返回所有圖片id
else:
print("Can not get the author's picture ids!")
exit()
獲取圖片的真實(shí)url并下載
訪問(wèn)url:https://www.pixiv.net/ajax/illust/圖片id?lang=zh,可以看到儲(chǔ)存有圖片真實(shí)地址的json:(這個(gè)json可以在圖片url:https://www.pixiv.net/artworks/圖片id 的開(kāi)發(fā)者面板中找到)
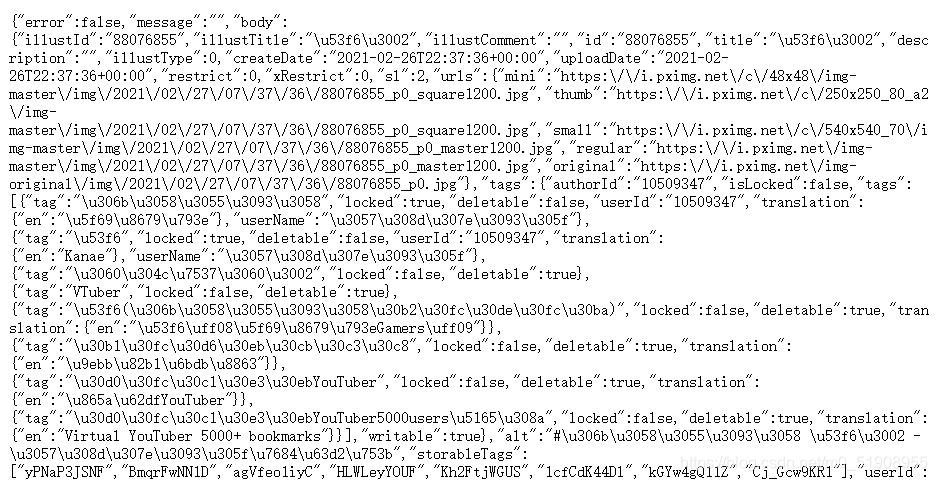
用同樣的方法提取json中有用的元素:
def getPictures(folder, IDlist, cookie): # 訪問(wèn)圖片儲(chǔ)存的真實(shí)網(wǎng)址
for picid in IDlist:
url1 = 'https://www.pixiv.net/artworks/{}'.format(picid) # 注意這里referer必不可少,否則會(huì)報(bào)403
headers = {
'User-Agent': user_agent,
'Cookie': cookie,
'Referer': url1
}
url = 'https://www.pixiv.net/ajax/illust/' + str(picid) + '?lang = zh' #訪問(wèn)儲(chǔ)存圖片網(wǎng)址的json
res = requests.get(url, headers=headers, proxies=proxies)
if res.status_code == 200:
data = json.loads(res.content)
picurl = data['body']['urls']['original'] # 在字典中找到儲(chǔ)存圖片的路徑與標(biāo)題
title = data['body']['title']
title = changeTitle(title) # 調(diào)整標(biāo)題
print(title)
print(picurl)
download(folder, picurl, title, headers)
else:
print("Can not get the urls of the pictures!")
exit()
def changeTitle(title): # 為了防止
global i
title = re.sub('[*:]', "", title) # 如果圖片中有下列符號(hào),可能會(huì)導(dǎo)致圖片無(wú)法成功下載
# 注意可能還會(huì)有許多不能用于文件命名的符號(hào),如果找到對(duì)應(yīng)符號(hào)要將其添加到正則表達(dá)式中
if title == '無(wú)題': # pixiv中有許多名為'無(wú)題'(日文)的圖片,需要對(duì)它們加以區(qū)分以防止覆蓋
title = title + str(i)
i = i + 1
return title
def download(folder, picurl, title, headers): # 將圖片下載到文件夾中
img = requests.get(picurl, headers=headers, proxies=proxies)
if img.status_code == 200:
with open(folder + '\\' + title + '.jpg', 'wb') as file: # 保存圖片
print("downloading:" + title)
file.write(img.content)
else:
print("download pictures error!")
完整代碼
import requests
from fake_useragent import UserAgent
import json
import re
import os
global i
i = 0
ua = UserAgent() # 生成假的瀏覽器請(qǐng)求頭,防止被封ip
user_agent = ua.random # 隨機(jī)選擇一個(gè)瀏覽器
proxies = {'http': 'http://127.0.0.1:51837', 'https': 'http://127.0.0.1:51837'} # 代理,根據(jù)自己實(shí)際情況調(diào)整,注意在請(qǐng)求時(shí)一定不要忘記代理!!
def makefolder(id): # 根據(jù)畫(huà)師的id創(chuàng)建對(duì)應(yīng)的文件夾
try:
folder = os.path.join('E:\pixivimages', id)
os.mkdir(folder)
return folder
except(FileExistsError):
print('the folder exists!')
exit()
def getAuthorAllPicID(id, cookie): # 獲取畫(huà)師所有圖片的id
url = 'https://pixiv.net/ajax/user/' + id + '/profile/all' # 訪問(wèn)存有畫(huà)師所有作品
headers = {
'User-Agent': user_agent,
'Cookie': cookie,
'Referer': 'https://www.pixiv.net/artworks/'
}
res = requests.get(url, headers=headers, proxies=proxies)
if res.status_code == 200:
resdict = json.loads(res.content)['body']['illusts'] # 將json轉(zhuǎn)化為python的字典后提取元素
return [key for key in resdict] # 返回所有圖片id
else:
print("Can not get the author's picture ids!")
exit()
def getPictures(folder, IDlist, cookie): # 訪問(wèn)圖片儲(chǔ)存的真實(shí)網(wǎng)址
for picid in IDlist:
url1 = 'https://www.pixiv.net/artworks/{}'.format(picid) # 注意這里referer必不可少,否則會(huì)報(bào)403
headers = {
'User-Agent': user_agent,
'Cookie': cookie,
'Referer': url1
}
url = 'https://www.pixiv.net/ajax/illust/' + str(picid) + '?lang = zh' #訪問(wèn)儲(chǔ)存圖片網(wǎng)址的json
res = requests.get(url, headers=headers, proxies=proxies)
if res.status_code == 200:
data = json.loads(res.content)
picurl = data['body']['urls']['original'] # 在字典中找到儲(chǔ)存圖片的路徑與標(biāo)題
title = data['body']['title']
title = changeTitle(title) # 調(diào)整標(biāo)題
print(title)
print(picurl)
download(folder, picurl, title, headers)
else:
print("Can not get the urls of the pictures!")
exit()
def changeTitle(title): # 為了防止
global i
title = re.sub('[*:]', "", title) # 如果圖片中有下列符號(hào),可能會(huì)導(dǎo)致圖片無(wú)法成功下載
# 注意可能還會(huì)有許多不能用于文件命名的符號(hào),如果找到對(duì)應(yīng)符號(hào)要將其添加到正則表達(dá)式中
if title == '無(wú)題': # pixiv中有許多名為'無(wú)題'(日文)的圖片,需要對(duì)它們加以區(qū)分以防止覆蓋
title = title + str(i)
i = i + 1
return title
def download(folder, picurl, title, headers): # 將圖片下載到文件夾中
img = requests.get(picurl, headers=headers, proxies=proxies)
if img.status_code == 200:
with open(folder + '\\' + title + '.jpg', 'wb') as file: # 保存圖片
print("downloading:" + title)
file.write(img.content)
else:
print("download pictures error!")
def main():
global i
id = input('input the id of the artist:')
cookie = input('input your cookie:') # 半自動(dòng)爬蟲(chóng),需要自己事先登錄pixiv以獲取cookie
folder = makefolder(id)
IDlist = getAuthorAllPicID(id, cookie)
getPictures(folder, IDlist, cookie)
if __name__ == '__main__':
main()
效果
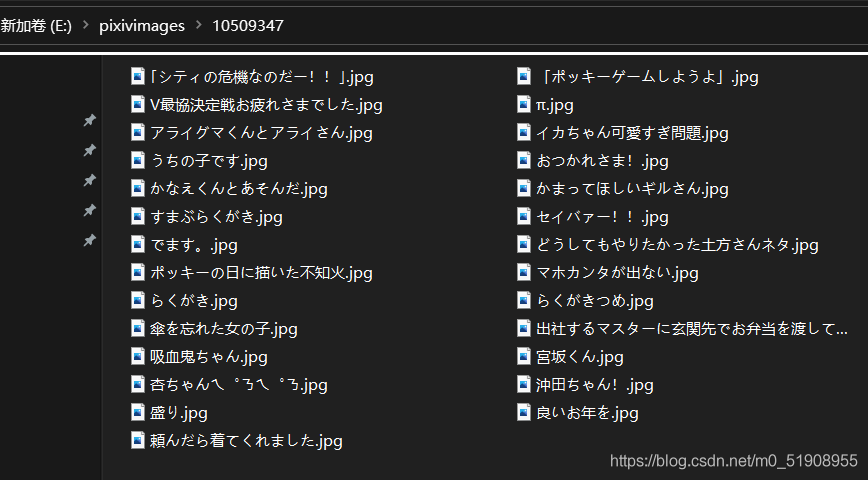
總結(jié)
到此這篇關(guān)于Python爬取動(dòng)態(tài)網(wǎng)頁(yè)中圖片的文章就介紹到這了,更多相關(guān)Python爬取動(dòng)態(tài)網(wǎng)頁(yè)圖片內(nèi)容請(qǐng)搜索腳本之家以前的文章或繼續(xù)瀏覽下面的相關(guān)文章希望大家以后多多支持腳本之家!
您可能感興趣的文章:- Python使用爬蟲(chóng)抓取美女圖片并保存到本地的方法【測(cè)試可用】
- 使用Python的Scrapy框架十分鐘爬取美女圖
- Python制作爬蟲(chóng)抓取美女圖
- python實(shí)現(xiàn)爬蟲(chóng)下載美女圖片
- python爬蟲(chóng)入門(mén)教程之點(diǎn)點(diǎn)美女圖片爬蟲(chóng)代碼分享
- python小技巧之批量抓取美女圖片
- Python爬蟲(chóng)之教你利用Scrapy爬取圖片
- python制作微博圖片爬取工具
- Python使用xpath實(shí)現(xiàn)圖片爬取
- 只用50行Python代碼爬取網(wǎng)絡(luò)美女高清圖片